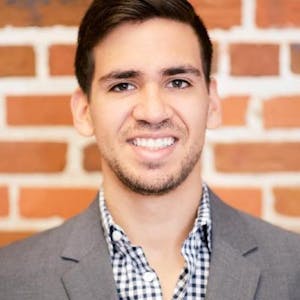
David Khourshid
David Khourshid (known on Twitter as David K. Piano) is a software engineer for Microsoft, a tech author, and speaker. Also a fervent open-source contributor, he is passionate about statecharts and software modeling, reactive animations, innovative user interfaces, and cutting-edge front-end technologies. When not behind a computer keyboard, he’s behind a piano keyboard or traveling.
Making State Management Intelligent
React Summit 2024

Jun 13, 22:00
Making State Management Intelligent
Managing state is complicated. Humans are even more complicated. As developers, it's our job to deliver seamless and intuitive user experiences, but the sheer complexity of human behavior and the real world can make this a daunting task. In this talk, we'll explore a radical new approach to app development where language models (LLMs) and reinforcement learning (RL) can be used to handle app logic in a more intelligent and human-centric way. We're bringing artificial intelligence to state management in ways that go much, much further than calling the ChatGPT API. You will learn how you can leverage AI in your existing code to create the best UX possible, and peer into the future of AI and the path to AGI.
Using useEffect Effectively
React Advanced Conference 2022

30 min
Using useEffect Effectively
Top ContentCan useEffect affect your codebase negatively? From fetching data to fighting with imperative APIs, side effects are one of the biggest sources of frustration in web app development. And let’s be honest, putting everything in useEffect hooks doesn’t help much. In this talk, we'll demystify the useEffect hook and get a better understanding of when (and when not) to use it, as well as discover how declarative effects can make effect management more maintainable in even the most complex React apps.
The State of XState
React Finland 2021

18 min
The State of XState
Over the past few years, state machines, statecharts, and the actor model have proven to be viable concepts for building complex application logic in a clear, visual way with XState. In this talk, we'll take a peek into the future of XState, including new features in the next version, and new tools and services that will make it even easier to create and collaborate on state machines.
The Visual Future of State Management
JSNation Live 2021

32 min
The Visual Future of State Management
Learn about state modeling with state machines and statecharts can improve the way you develop application logic, and get a sneak peek of never-before-seen upcoming visual tools that will take state management to the next level.
XState: the Visual Future of State Management
React Summit Remote Edition 2021

35 min
XState: the Visual Future of State Management
Top ContentLearn about state modeling with state machines and statecharts can improve the way you develop your React applications, and get a sneak peek of never-before-seen upcoming visual tools that will take state management to the next level.